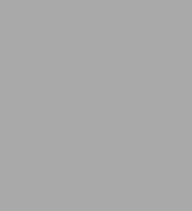
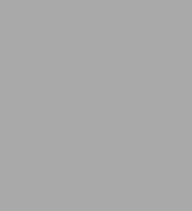
eBook
Related collections and offers
Overview
CodeNotes for C# illustrates Microsoft’s new language for the .NET platform, emphasizing syntax features and object-oriented concepts. Major capabilities of the .NET platform, including the Common Language Runtime (CLR), Base Class Libraries (BCL), and Assemblies, are also covered, as are popular .NET services such as ADO.NET, web services, and ASP.NET. This book is aimed at the experienced developer (Java, VB, C++, etc.) who wants to learn the C# language and evaluate its new features.
This edition of CodeNotes includes:
• A global overview of this technology and explanation of what problems it can be used to solve
• Real-world examples
• “How and Why” sections that provide hints, tricks, workarounds, and tips on what should be taken advantage of or avoided
• Instructions and classroom-style tutorials throughout from expert trainers and software developers
Product Details
ISBN-13: | 9781588362643 |
---|---|
Publisher: | Random House Publishing Group |
Publication date: | 12/10/2002 |
Series: | CodeNotes |
Sold by: | Random House |
Format: | eBook |
Pages: | 272 |
File size: | 840 KB |
About the Author
Read an Excerpt
Chapter 1
INTRODUCTION
Innovation is the hallmark of the software industry. Like clockwork, the widespread adoption of a particular standard is frequently accompanied by the introduction of a new one. Programming languages are no exception. Drawing upon both theory and practice, a new language will often address the shortcomings and limitations of its predecessors while boasting innovations to boot. So it is with C#.
As you have likely heard, C# (pronounced "C-Sharp") is a new programming language designed by Microsoft. Although the spectrum of existing documentation on C# can give varying impressions as to its place within the software development community, a diagnosis of the language is prone to the following two distinct observations:
1. Formally, C# is a specification for a computer language that Microsoft announced in June 2000. Syntactically, the language is similar to C++ and Java, eliminating (or obscuring) many of the complexities of the former while improving on some of the conventions of the latter. The C# specification was recently ratified by the European Computer Manufacturers Association (ECMA), which means that other vendors can provide their own implementations of the language. Therefore, writing C# applications for operating systems other than Windows will be possible (in fact, as we will see, it is already possible).
2. Practically, C# is the "native" language of the .NET Framework, which is Microsoft's new strategy for the development and deployment of software. As we will see throughout this book, in addition to revamping significant portions of Windows architecture, .NET improves upon existing Microsoft technologies such as ActiveX Data Objects (ADO, for database access) and Active Server Pages (ASP, for web development). Furthermore, .NET introduces some fundamentally new concepts into the realm of software development, such as cross-language inheritance and component versioning through public-key cryptography.
In this regard, C# is not so much a new creature as an improved form of older languages that has been adapted to the .NET environment. For developers C# can serve as an excellent entry point into the .NET world (although, as we will see, other languages are also supported by the framework). By using C# to leverage the .NET Framework you can develop a variety of applications, including traditional Windows desktop applications, web-based applications, and software components that communicate over the open standards of the Internet (web services).
In this book we will examine both the theory and practice behind C#. Whereas theory encompasses the syntactical conventions of the language (such as supported variable types, loop constructs, and object-oriented features), practice incorporates the surrounding .NET Framework on which a C# application executes. Remember, however, that technically C# is nothing more than language specification, and while in practice it is intrinsically tied to .NET, there is no stipulation that it remain so. (An open source effort to port the language to Linux is already in progress; see aCS010001 for details.) Nevertheless, a portion of this book is dedicated to exploring the natural habitat of C#, which is .NET.
C# Is Just Another Language . . .
While it is easy to become buried in the marketing hyperbole, remember that C# is just another language. Although there are some noteworthy innovations with Microsoft's latest creature, the language merely builds on concepts with which you are likely familiar. Memory allocation, array usage, and class inheritance are all-important aspects of C#, though they may be used with slightly different conventions and assumptions than the language you are currently using.
What is special about C# is that it was designed specifically with component development in mind. This means that authoring classes in C# is extremely straightforward because of the language's intrinsic support for component-based features such as properties and events (in contrast to existing languages that obscure the process to varying degrees).
From a syntax perspective, C# is a derivative of the "C" language family, which means that C++ and Java developers will be able to pick it up with relative ease. The language will pose more of a challenge to Visual Basic developers because its syntax is not as rich or "English-like." In addition, C# is built on object-oriented (OO) features such as inheritance, polymorphism, and interfaces, which were either absent or obscured in Visual Basic 6. In the upcoming orientation section we will expand on some of the challenges that developers will face as they migrate from a particular language to C#.
. . . and a Completely New Framework
C# may be just another language, but it is built on a fundamentally new framework. It can be argued that .NET represents the most significant architectural change in Microsoft technology since the introduction of 32-bit Windows 95. Many of the concepts and technologies that you may be familiar with, such as DLLs, COM, and ActiveX, have been overhauled or deprecated by .NET. (As we will see, however, they have not been rendered obsolete because there is still a place for legacy technologies within the framework.) In addition, .NET applications are not run directly by the operating system but instead execute through an intermediary called the Common Language Runtime (CLR), which in some ways is similar to a Java Virtual Machine (VM).
Two Steps
Given these two aspects of Microsoft's new development tool, the requirements for becoming a productive C# developer are twofold:
1. You must familiarize yourself with the conventions of the language. This includes understanding how C# syntax elements (classes, functions, loops, etc.) are utilized as well as learning how programmatic tasks (such as the manipulation of strings) are carried out.
2. You must understand the intricacies of the .NET Framework. To this end let us now examine exactly what the .NET Framework is.
THE .NET FRAMEWORK
C# is simply a piece of a larger and more encompassing entity: Microsoft's new .NET Framework. Depending on your interests and development background, you may already have a number of ideas as to what the .NET Framework entails. As we will see in this book:
* .NET fundamentally changes the manner in which applications execute under the Windows Operating System. With .NET, Microsoft is in effect abandoning its traditional stance, which favors compiled components, and is embracing Virtual Machine (VM) technology (similar in many ways to the Java paradigm).
* In addition to introducing C#, .NET brings about significant changes to both Visual Basic and Visual C++. .NET also provides a common library for all languages (discussed shortly).
* .NET is built from the ground up with the Internet in mind and embraces open Internet standards such as XML and HTTP. XML is also used throughout the framework as a messaging instrument, for file storage configuration, and for documentation purposes in C#.
These are all noteworthy features of the .NET Framework, which consists of the platform and tools needed to develop and deploy .NET applications. We will take a closer look at the .NET Framework in Chapter 3, but its essential components are summarized here. For a thorough introduction, however, please see CodeNotes for .NET.
The Common Language Runtime
The Common Language Runtime (CLR) is the execution engine for all programs in the .NET Framework. The CLR is similar to a Java Virtual Machine (VM) in that it executes byte code on the fly while simultaneously providing services such as garbage collection and exception handling. Unlike a Java VM, which is limited to the Java language, the CLR is accessible from any compiler that produces Microsoft Intermediate Language (IL) code, which is similar to Java byte code. Code that executes within the CLR is referred to as "managed" code. Code that executes outside its boundaries (such as VB6 and Win32 applications) is called "unmanaged" code. The C# compiler does not translate source code into native machine code but converts it into IL code, which is, in turn, run by the CLR. Therefore, C# applications are said to be "managed" by the CLR.
The Base Class Libraries
In C# (and in .NET), functionality is exposed through a collection of classes called the Base Class Libraries (BCL). The BCL provides thousands of prewritten classes that you can leverage for services such as File I/O, database access, and common operations such as sorting. Consider, for example, how you currently manipulate a string. The approach depends, of course, on the language you use:
* Visual Basic users would leverage built-in language functions such as Mid$(), InStr$(), etc.
* C++ developers could manipulate the string directly in memory (using pointers) or, more likely, leverage prewritten libraries such as the C-Runtime Library, the Standard Template Library (STL), or the Microsoft Foundation Classes (MFC).
* Those using Java would utilize the String or StringBuffer class of the Java Class Libraries (JCL). (Java users will find the BCL very similar to the JCL.)
In C# you manipulate strings by using the System.String and System.StringBuilder classes found in the BCL. The BCL is significant because it is accessible to any language that targets the CLR (not just C#). This is in contrast to pre-.NET technology where every language has its own supporting libraries that are accessible only from that particular language (the MFC, for example, is only accessible from C++). Thus, once you understand how to use the System.String class, you can leverage it not only from C# but from any of the .NET languages discussed below.
C# Compiler Tools
Writing .NET applications requires a compiler that translates source code into IL code. There are two ways to perform the compilation process in C#:
1. Using the C# command-line compiler (csc.exe) that ships with the .NET Framework. The command-line compiler allows you to write C# code in a text editor (Notepad, for example) and then produce a .NET program from the command prompt.
2. Using Microsoft's latest Integrated Development Environment (IDE)-Visual Studio .NET (VS.NET for short). Visual Studio .NET is an amalgamation of the Visual Studio 6 environment that was used for Visual C++ and the intuitive VB Forms edi-tor that was used for Visual Basic development. VS.NET offers many sophisticated features such as automatic code generation, powerful debugging capabilities, and Rapid Application Development (RAD) facilities.
We will illustrate both compilation approaches at the end of this chapter, but in general you will want to use VS.NET for C# development. As we will see, for certain operations the IDE generates a considerable amount of boilerplate code behind the scenes, which you would have to write manually if you developed outside the tool's confines. However, unlike the command-line compiler that ships with the .NET Framework (which is available from Microsoft for free), Visual Studio .NET must be purchased separately.
In addition to language compilers, the .NET Framework contains a large assortment of command-line utilities. Some of these utilities are incorporated directly in VS.NET's development environment, whereas others are exclusively stand-alone. We will examine some of these utilities throughout this book.
The .NET Languages
In addition to C#, Microsoft provides four other language compilers for the .NET Framework:
1. Visual Basic.NET, the new version of Visual Basic
2. JScript.NET, an evolved version of the popular scripting tool
3. Managed C++, a modified version of C++
4. J#, Microsoft's offering of the Java syntax for the .NET Framework
The first two compilers ship as part of the framework itself (and thus are free), the third is included with VS.NET, and the fourth must be purchased separately. Other companies are in the process of porting additional languages to the .NET Framework. Two noteworthy efforts are NetCOBOL by Fujitsu Software and Visual Perl by Active State. For more information see aCS010002.
Although the focus of this book is obviously C#, it helps to understand the other languages in the .NET Framework because language interoperability is a cornerstone of .NET development.
Visual Basic.NET
When Visual Basic was first released in 1992, its approach to application design was remarkably straightforward. Instead of the C++ technique, which required many lines of complicated code, VB allowed developers to "paint" their applications within a comfortable design environment. This approach was not only more intuitive than C++ but much faster as well. Even C++ purists had to concede that what was arduous in C++ was easier in VB. Although Visual Basic's capabilities now transcend GUI design, it is still a popular choice for creating desktop applications for the Windows Operating System.
As its name suggests, Visual Basic.NET is the latest version of Visual Basic for the .NET Framework. Under this new version of the product (which was once known as Visual Basic 7), the language has undergone a significant face-lift. In addition to introducing new features into the language (such as true object inheritance and structured exception handling), VB.NET has removed some cumbersome and unusual aspects of its predecessor (e.g., default properties, optional parentheses, etc.). As a result, VB.NET brings about some syntax changes that break compatibility with old VB source code. For example, procedure parameters are now passed by value (ByVal) by default, and not by reference (ByRef). Certain syntax elements such as GoSub, IsNull, and IsMissing have been removed from the language altogether. For a thorough introduction to VB.NET, see CodeNotes for VB.NET.